Luxtelligence provides a library of components that have been fabricated in the reference material stack, and whose performance has been tested and validated. Here follows a list of the available parametric cells (gdsfactory.Component objects):
Cells#
CPW_pad_linear#
- lnoi400.cells.CPW_pad_linear(start_width: float = 80.0, length_straight: float = 10.0, length_tapered: float = 190.0, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_uni_cpw') Component [source]#
RF access line for high-frequency GSG probes. The probe pad maintains a fixed gap/central conductor ratio across its length, to achieve a good impedance matching
import lnoi400
c = lnoi400.cells.CPW_pad_linear(start_width=80.0, length_straight=10.0, length_tapered=190.0, cross_section='xs_uni_cpw')
c.plot()
(Source code
, png
, hires.png
, pdf
)
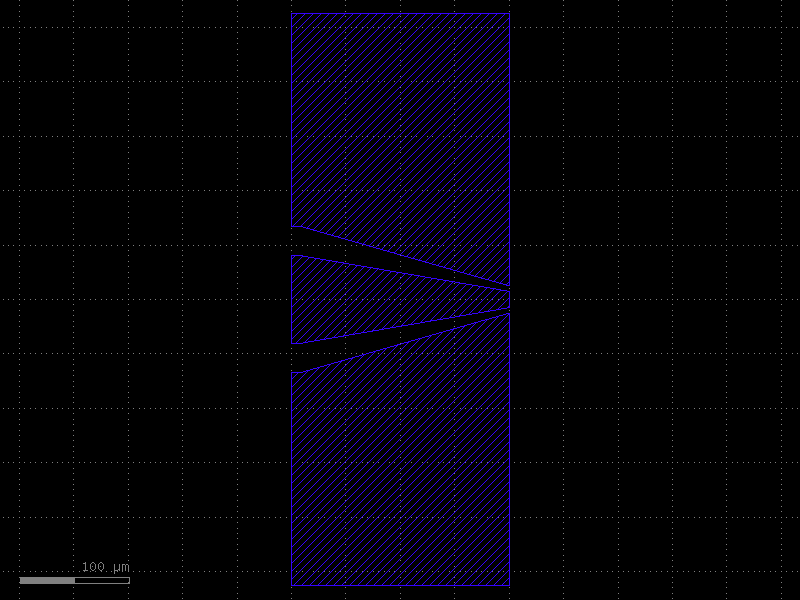
L_turn_bend#
- lnoi400.cells.L_turn_bend(radius: float = 80.0, p: float = 1.0, with_arc_floorplan: bool = True, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg1000', **kwargs) Component [source]#
A 90-degrees bend following an Euler path, with linearly-varying curvature (increasing and decreasing).
import lnoi400
c = lnoi400.cells.L_turn_bend(radius=80.0, p=1.0, with_arc_floorplan=True, cross_section='xs_rwg1000')
c.plot()
(Source code
, png
, hires.png
, pdf
)
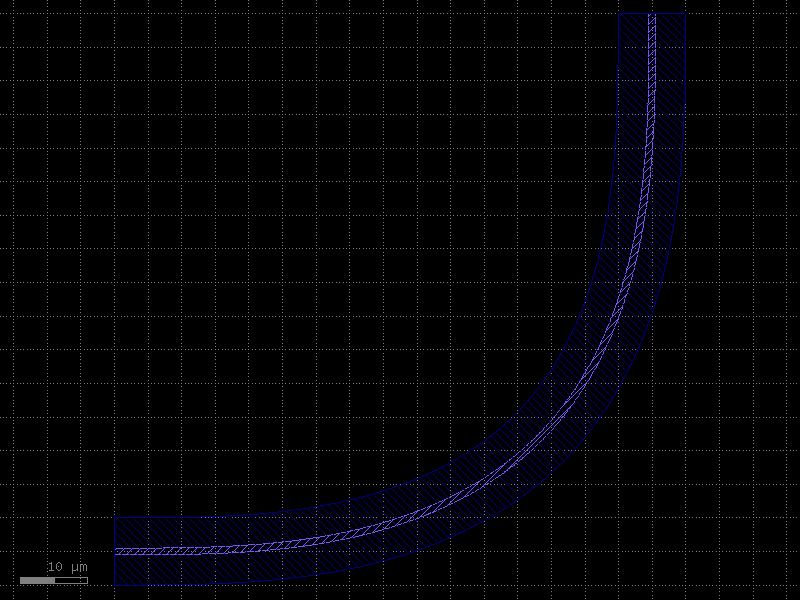
S_bend_vert#
- lnoi400.cells.S_bend_vert(v_offset: float = 25.0, h_extent: float = 100.0, dx_straight: float = 5.0, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg1000') Component [source]#
A spline bend that bridges a vertical displacement.
import lnoi400
c = lnoi400.cells.S_bend_vert(v_offset=25.0, h_extent=100.0, dx_straight=5.0, cross_section='xs_rwg1000')
c.plot()
(Source code
, png
, hires.png
, pdf
)
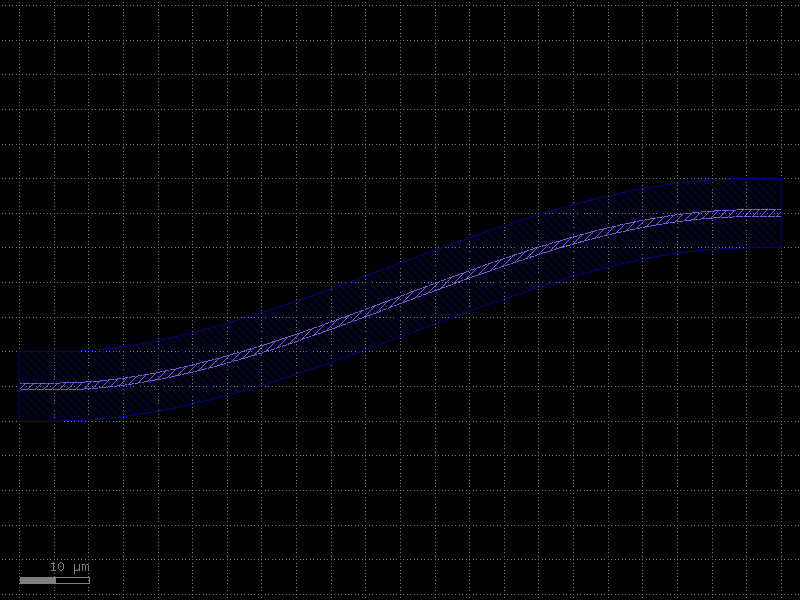
U_bend_racetrack#
- lnoi400.cells.U_bend_racetrack(v_offset: float = 90.0, p: float = 1.0, with_arc_floorplan: bool = True, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg3000', **kwargs) Component [source]#
A U-bend with fixed cross-section and dimensions, suitable for building a low-loss racetrack resonator.
import lnoi400
c = lnoi400.cells.U_bend_racetrack(v_offset=90.0, p=1.0, with_arc_floorplan=True, cross_section='xs_rwg3000')
c.plot()
(Source code
, png
, hires.png
, pdf
)
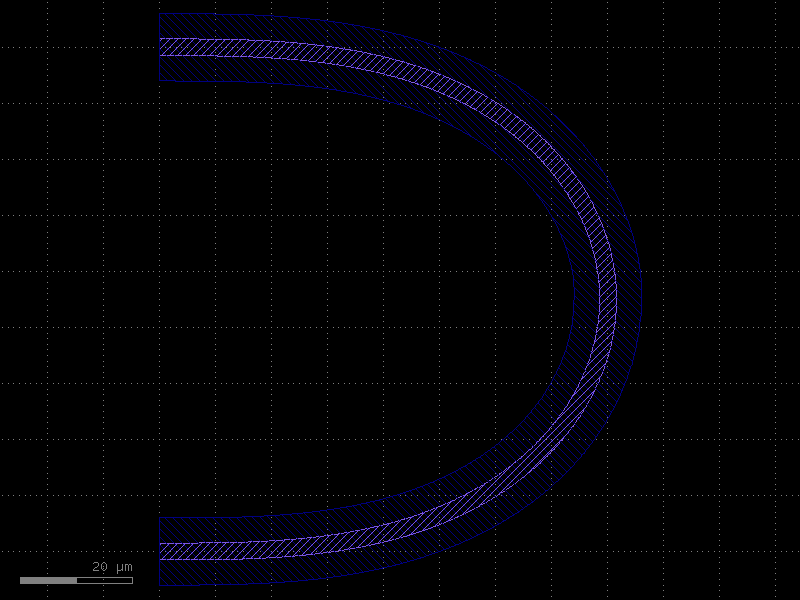
bend_S_spline#
- lnoi400.cells.bend_S_spline(size: tuple[float, float] = (100.0, 30.0), cross_section: ~collections.abc.Callable[[...], ~gdsfactory.cross_section.CrossSection] | ~gdsfactory.cross_section.CrossSection | dict[str, ~typing.Any] | str | ~gdsfactory.cross_section.Transition = 'xs_rwg1000', npoints: int = 201, path_method=<function spline_clamped_path>) Component [source]#
A spline bend merging a vertical offset.
import lnoi400
c = lnoi400.cells.bend_S_spline(size=(100.0, 30.0), cross_section='xs_rwg1000', npoints=201)
c.plot()
(Source code
, png
, hires.png
, pdf
)
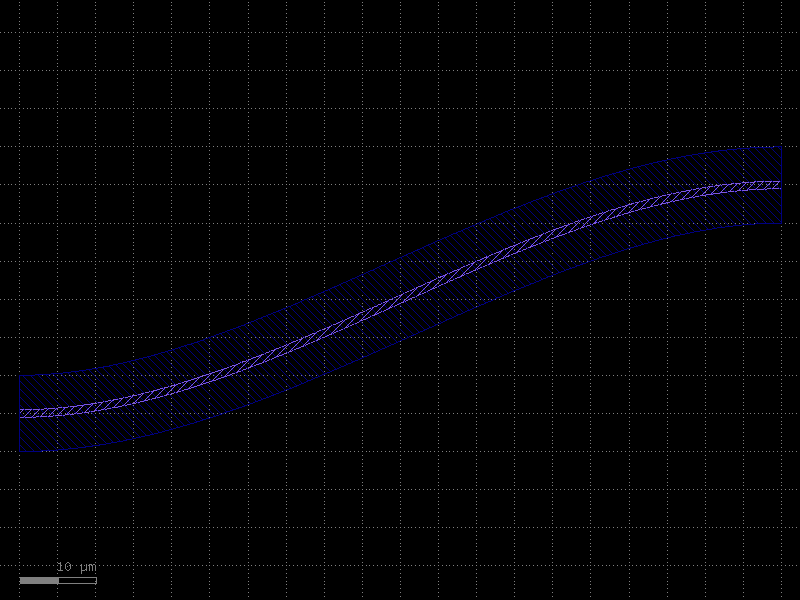
bend_S_spline_varying_width#
- lnoi400.cells.bend_S_spline_varying_width(size: tuple[float, float] = (58, 14.5), cross_section1: ~collections.abc.Callable[[...], ~gdsfactory.cross_section.CrossSection] | ~gdsfactory.cross_section.CrossSection | dict[str, ~typing.Any] | str | ~gdsfactory.cross_section.Transition = None, cross_section2: ~collections.abc.Callable[[...], ~gdsfactory.cross_section.CrossSection] | ~gdsfactory.cross_section.CrossSection | dict[str, ~typing.Any] | str | ~gdsfactory.cross_section.Transition = None, npoints: int = 201, path_method=<function spline_null_curvature>) Component [source]#
A spline bend merging a vertical offset. Can accept arbitrary cross sections. Not tested as a standalone PDK element. Used as a building block for cells with known behaviour.
import lnoi400
c = lnoi400.cells.bend_S_spline_varying_width(size=(58, 14.5), npoints=201)
c.plot()
(Source code
, png
, hires.png
, pdf
)
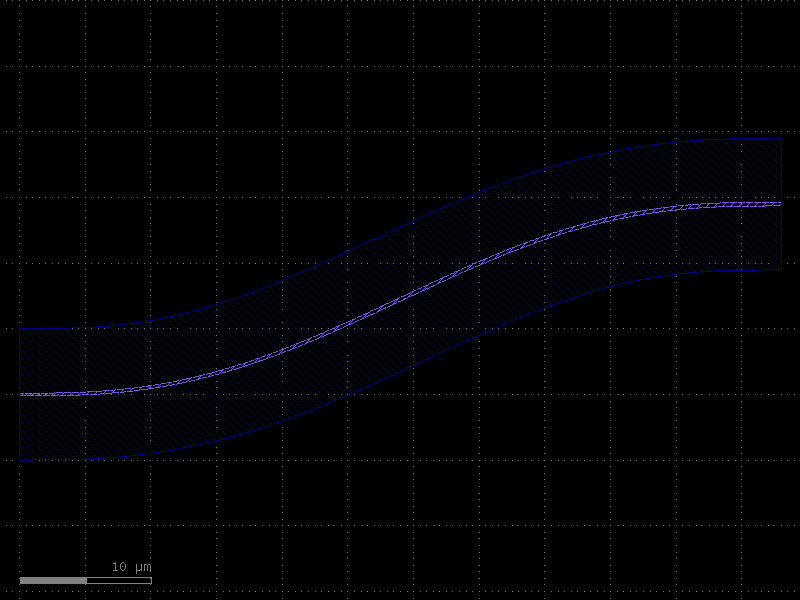
chip_frame#
- lnoi400.cells.chip_frame(size: tuple[float, float] = (10000, 5000), exclusion_zone_width: float = 50, center: tuple[float, float] = None) Component [source]#
Provide the chip extent and the exclusion zone around the chip frame. In the exclusion zone, only the edge couplers routing to the chip facet should be placed. Allowed chip dimensions (in either direction): 5000 um, 10000 um, 20000 um.
import lnoi400
c = lnoi400.cells.chip_frame(size=(10000, 5000), exclusion_zone_width=50)
c.plot()
(Source code
, png
, hires.png
, pdf
)
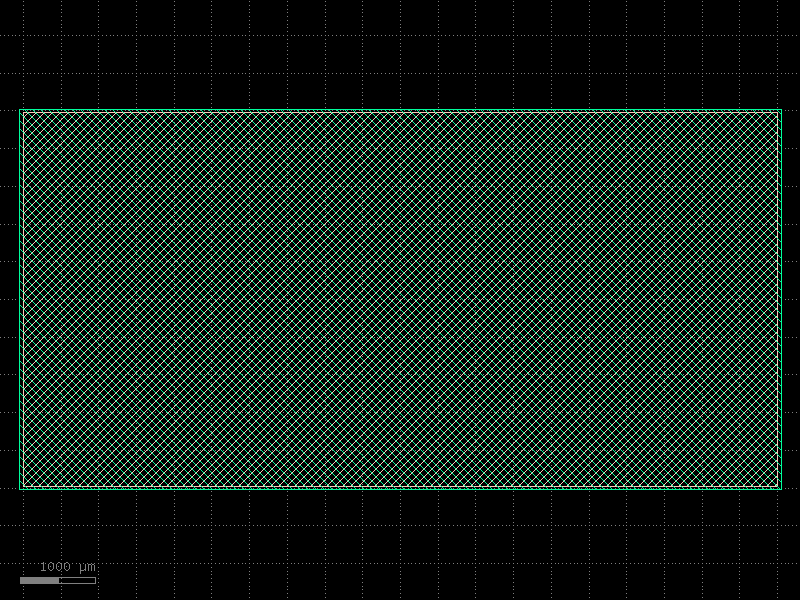
directional_coupler_balanced#
- lnoi400.cells.directional_coupler_balanced(io_wg_sep: float = 30.6, sbend_length: float = 58, central_straight_length: float = 16.92, coupl_wg_sep: float = 0.8, coup_wg_width: float = 0.8, cross_section_io: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg1000') Component [source]#
Returns a 50-50 directional coupler. Default parameters give a 50/50 splitting at 1550 nm.
- Parameters:
io_wg_sep – Separation of the two straights at the input/output, top-to-top.
sbend_length – length of the s-bend part.
central_straight_length – length of the coupling region.
coupl_wg_sep – Distance between two waveguides in the coupling region (side to side).
cross_section_io – cross section spec at the i/o (must be defined in tech.py).
coup_wg_width – waveguide width at the coupling section.
import lnoi400
c = lnoi400.cells.directional_coupler_balanced(io_wg_sep=30.6, sbend_length=58, central_straight_length=16.92, coupl_wg_sep=0.8, coup_wg_width=0.8, cross_section_io='xs_rwg1000')
c.plot()
(Source code
, png
, hires.png
, pdf
)
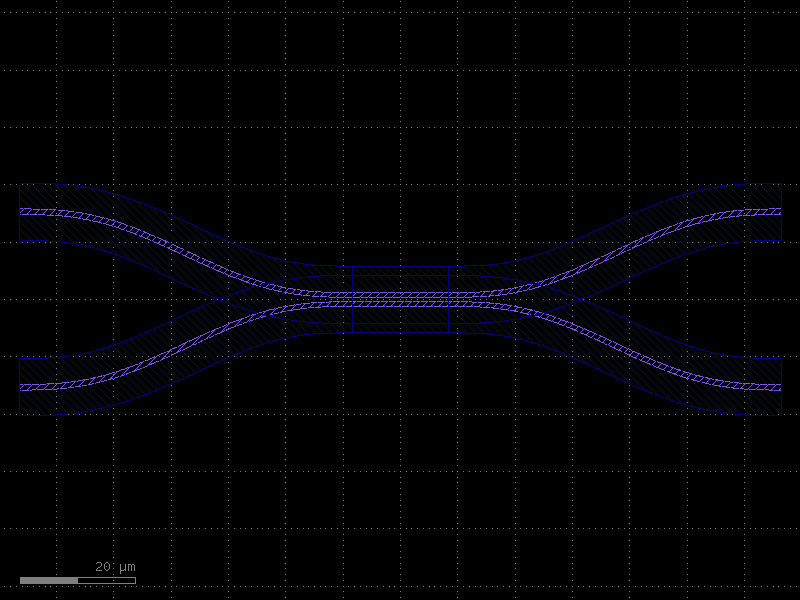
double_linear_inverse_taper#
- lnoi400.cells.double_linear_inverse_taper(cross_section_start: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_swg250', cross_section_end: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg1000', lower_taper_length: float = 120.0, lower_taper_end_width: float = 2.05, upper_taper_start_width: float = 0.25, upper_taper_length: float = 240.0, slab_removal_width: float = 20.0, input_ext: float = 0.0) Component [source]#
Inverse taper with two layers, starting from a wire waveguide at the facet and transitioning to a rib waveguide. The tapering profile is linear in both layers.
import lnoi400
c = lnoi400.cells.double_linear_inverse_taper(cross_section_start='xs_swg250', cross_section_end='xs_rwg1000', lower_taper_length=120.0, lower_taper_end_width=2.05, upper_taper_start_width=0.25, upper_taper_length=240.0, slab_removal_width=20.0, input_ext=0.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
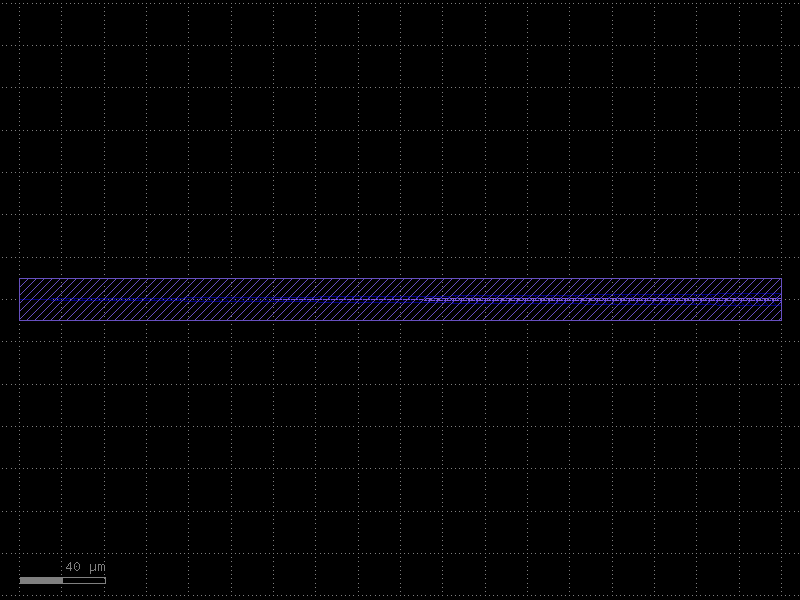
eo_phase_shifter#
- lnoi400.cells.eo_phase_shifter(rib_core_width_modulator: float = 2.5, taper_length: float = 100.0, modulation_length: float = 7500.0, rf_central_conductor_width: float = 10.0, rf_ground_planes_width: float = 180.0, rf_gap: float = 4.0, draw_cpw: bool = True) Component [source]#
Phase shifter based on the Pockels effect. The waveguide is located within the gap of a CPW transmission line.
import lnoi400
c = lnoi400.cells.eo_phase_shifter(rib_core_width_modulator=2.5, taper_length=100.0, modulation_length=7500.0, rf_central_conductor_width=10.0, rf_ground_planes_width=180.0, rf_gap=4.0, draw_cpw=True)
c.plot()
(Source code
, png
, hires.png
, pdf
)
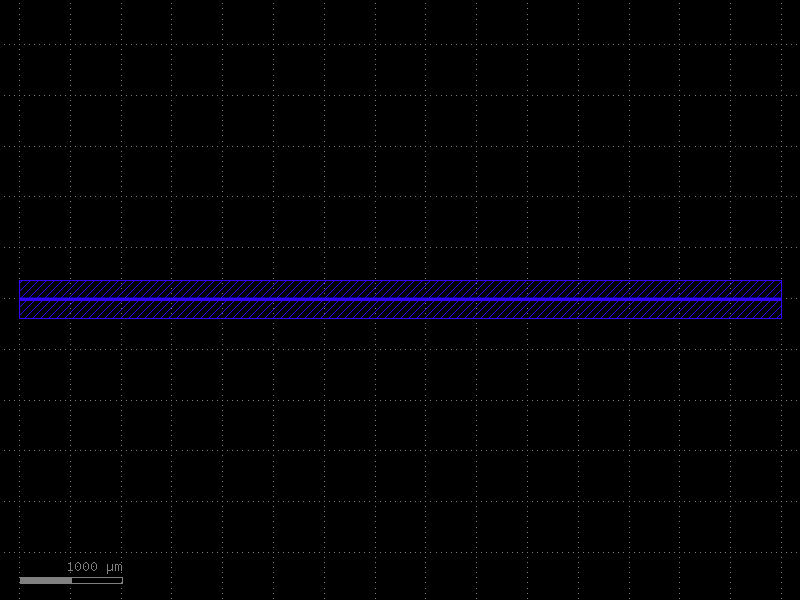
heater_resistor#
- lnoi400.cells.heater_resistor(path: Path | None = None, width: float = 0.9, offset: float = 0.0) Component [source]#
A resistive wire used as a low-frequency phase shifter, exploiting the thermo-optical effect.
import lnoi400
c = lnoi400.cells.heater_resistor(width=0.9, offset=0.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
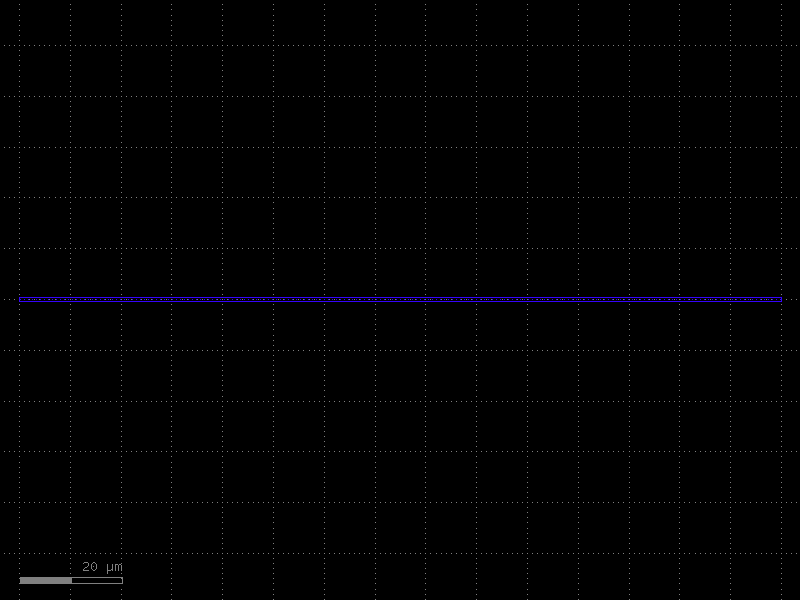
heater_straight_single#
- lnoi400.cells.heater_straight_single(length: float = 150.0, width: float = 0.9, offset: float = 0.0, port_contact_width_ratio: float = 3.0, pad_size: tuple[float, float] = (100.0, 100.0), pad_pitch: float | None = None, pad_vert_offset: float = 10.0) Component [source]#
A straight resistive wire used as a low-frequency phase shifter, exploiting the thermo-optical effect. The heater is terminated by wide pads for probing or bonding.
import lnoi400
c = lnoi400.cells.heater_straight_single(length=150.0, width=0.9, offset=0.0, port_contact_width_ratio=3.0, pad_size=(100.0, 100.0), pad_vert_offset=10.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
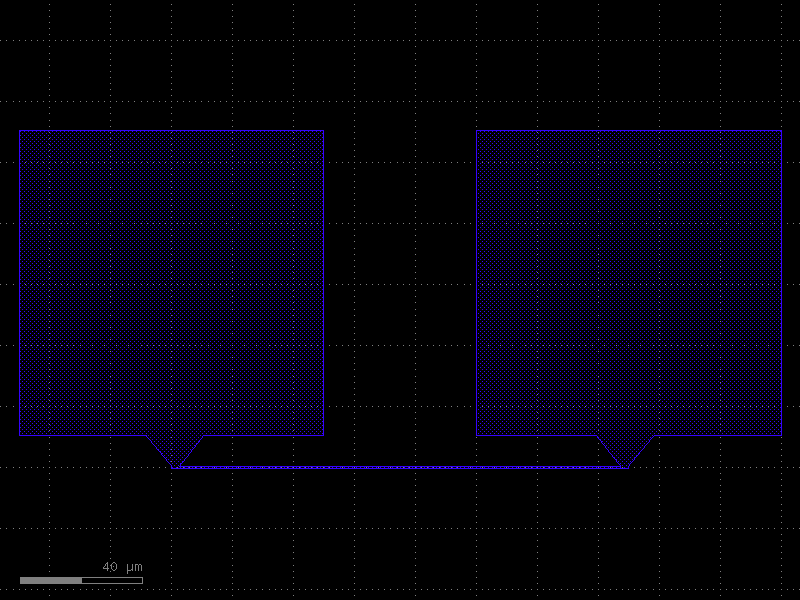
mmi1x2_optimized1550#
- lnoi400.cells.mmi1x2_optimized1550(width_mmi: float = 6.0, length_mmi: float = 26.75, width_taper: float = 1.5, length_taper: float = 25.0, port_ratio: float = 0.55, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg1000', **kwargs) Component [source]#
MMI1x2 with layout optimized for maximum transmission at 1550 nm.
import lnoi400
c = lnoi400.cells.mmi1x2_optimized1550(width_mmi=6.0, length_mmi=26.75, width_taper=1.5, length_taper=25.0, port_ratio=0.55, cross_section='xs_rwg1000')
c.plot()
(Source code
, png
, hires.png
, pdf
)
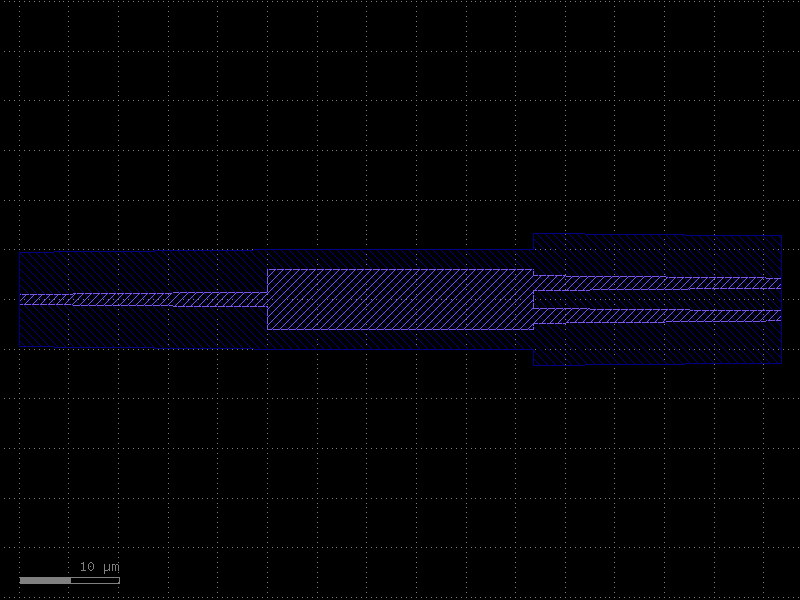
mmi2x2_optimized1550#
- lnoi400.cells.mmi2x2_optimized1550(width_mmi: float = 5.0, length_mmi: float = 76.5, width_taper: float = 1.5, length_taper: float = 25.0, port_ratio: float = 0.7, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg1000', **kwargs) Component #
MMI2x2 with layout optimized for maximum transmission at 1550 nm.
import lnoi400
c = lnoi400.cells.mmi2x2_optimized1550(width_mmi=5.0, length_mmi=76.5, width_taper=1.5, length_taper=25.0, port_ratio=0.7, cross_section='xs_rwg1000')
c.plot()
(Source code
, png
, hires.png
, pdf
)
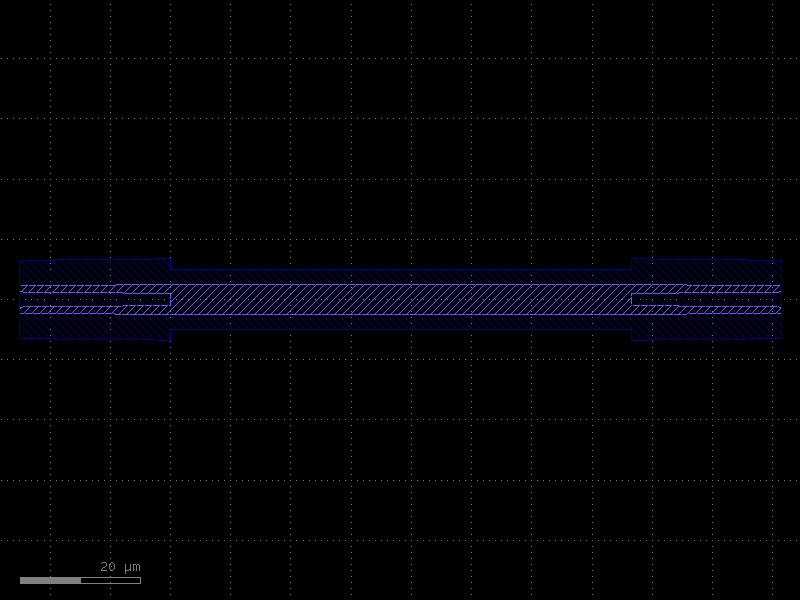
mmi2x2optimized1550#
- lnoi400.cells.mmi2x2optimized1550(width_mmi: float = 5.0, length_mmi: float = 76.5, width_taper: float = 1.5, length_taper: float = 25.0, port_ratio: float = 0.7, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_rwg1000', **kwargs) Component [source]#
MMI2x2 with layout optimized for maximum transmission at 1550 nm.
import lnoi400
c = lnoi400.cells.mmi2x2optimized1550(width_mmi=5.0, length_mmi=76.5, width_taper=1.5, length_taper=25.0, port_ratio=0.7, cross_section='xs_rwg1000')
c.plot()
(Source code
, png
, hires.png
, pdf
)
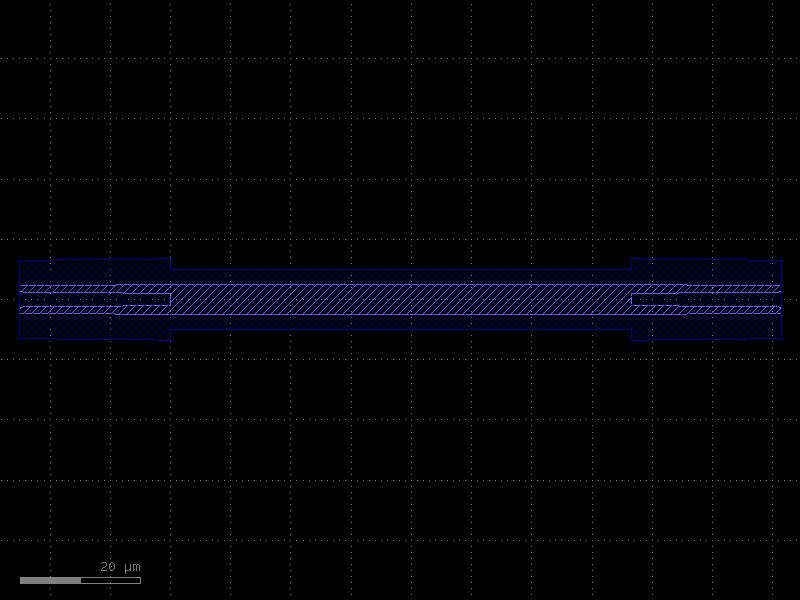
mzm_unbalanced#
- lnoi400.cells.mzm_unbalanced(modulation_length: float = 7500.0, length_imbalance: float = 100.0, lbend_tune_arm_reff: float = 75.0, rf_pad_start_width: float = 80.0, rf_central_conductor_width: float = 10.0, rf_ground_planes_width: float = 180.0, rf_gap: float = 4.0, rf_pad_length_straight: float = 10.0, rf_pad_length_tapered: float = 190.0, bias_tuning_section_length: float = 700.0, with_heater: bool = False, heater_offset: float = 1.2, heater_width: float = 1.0, heater_pad_size: tuple[float, float] = (75.0, 75.0), **kwargs) Component [source]#
Mach-Zehnder modulator based on the Pockels effect with an applied RF electric field. The modulator works in a differential push-pull configuration driven by a single GSG line.
import lnoi400
c = lnoi400.cells.mzm_unbalanced(modulation_length=7500.0, length_imbalance=100.0, lbend_tune_arm_reff=75.0, rf_pad_start_width=80.0, rf_central_conductor_width=10.0, rf_ground_planes_width=180.0, rf_gap=4.0, rf_pad_length_straight=10.0, rf_pad_length_tapered=190.0, bias_tuning_section_length=700.0, with_heater=False, heater_offset=1.2, heater_width=1.0, heater_pad_size=(75.0, 75.0))
c.plot()
(Source code
, png
, hires.png
, pdf
)
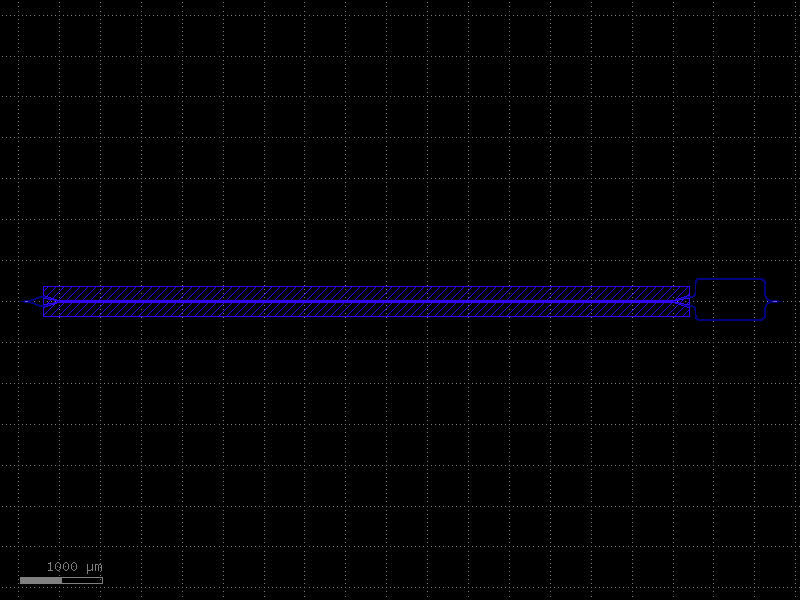
straight_rwg1000#
- lnoi400.cells.straight_rwg1000(length: float = 10.0, **kwargs) Component [source]#
Straight single-mode waveguide.
import lnoi400
c = lnoi400.cells.straight_rwg1000(length=10.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
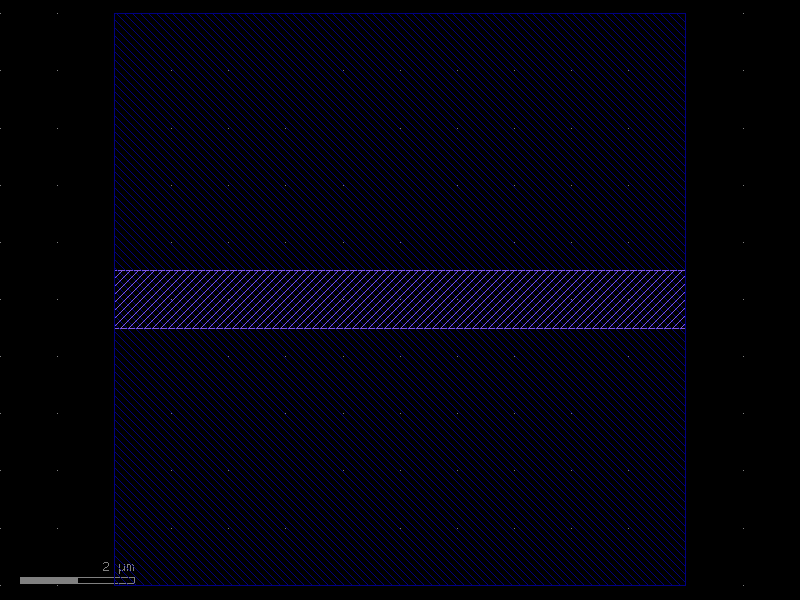
straight_rwg3000#
- lnoi400.cells.straight_rwg3000(length: float = 10.0, **kwargs) Component [source]#
Straight multimode waveguide.
import lnoi400
c = lnoi400.cells.straight_rwg3000(length=10.0)
c.plot()
(Source code
, png
, hires.png
, pdf
)
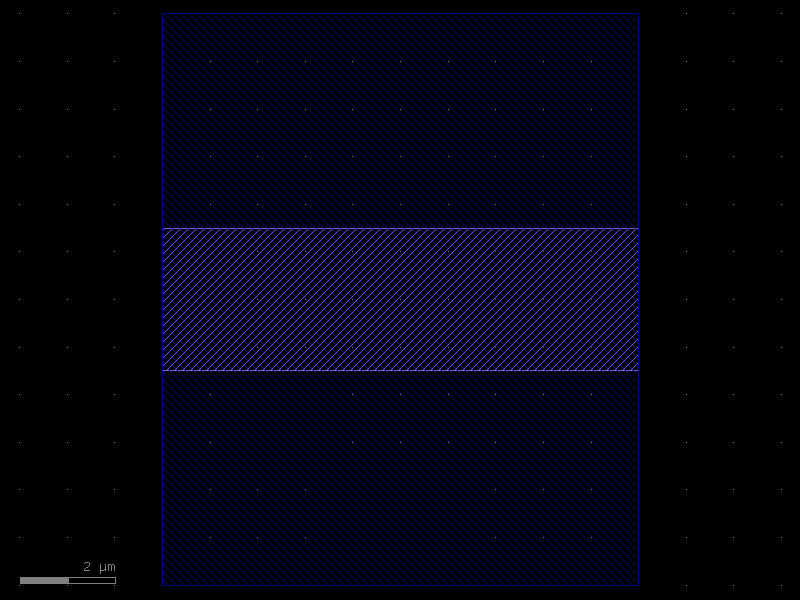
uni_cpw_straight#
- lnoi400.cells.uni_cpw_straight(length: float = 3000.0, cross_section: Callable[[...], CrossSection] | CrossSection | dict[str, Any] | str | Transition = 'xs_uni_cpw', bondpad: str | Callable[[...], Component] | dict[str, Any] | KCell = 'CPW_pad_linear') Component [source]#
A CPW transmission line for microwaves, with a uniform cross section.
import lnoi400
c = lnoi400.cells.uni_cpw_straight(length=3000.0, cross_section='xs_uni_cpw', bondpad='CPW_pad_linear')
c.plot()
(Source code
, png
, hires.png
, pdf
)
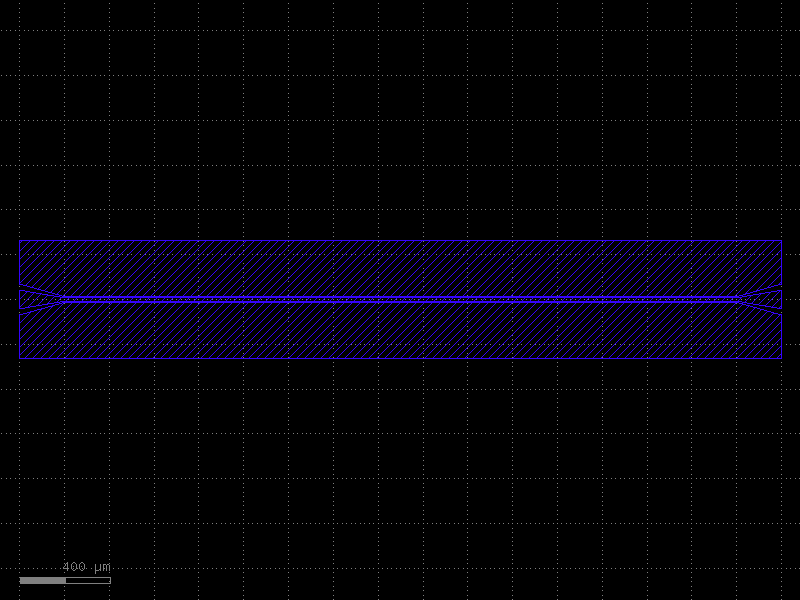